Arrays – 50 course points
The purpose of this assignment is to practice array manipulation.
Refer to our Programming Assignments FAQ for instructions on how to install VScode, how to use the command line and how to submit your assignments.
Programming
Write 2 programs and submit on Autolab.
- We provide a zip file (find it under BusStop on Autolab) containing BusStop.java and StaircaseBuilder.java.
- For each problem UPDATE and SUBMIT the corresponding file.
Observe the following rules:
- DO NOT add any import statements
- DO NOT add the project statement
- DO NOT change the class name
- DO NOT change the headers of ANY of the given methods
- DO NOT add any new class fields
- DO NOT use System.exit()
- ONLY print the result as specified by each problem. Observe the examples’ output, display only what the problem is asking for.
- DO NOT print other messages, follow the examples for each problem.
- RU BusStop (25 points). Serena, a freshmen at Rutgers just finished her Intro to Logic class on College Ave and needs to return to Busch for her Intro to Computer Science class. She is currently standing at the Yard Bus Stop, waiting patiently for the H bus to arrive. Your job is to help Serena find in which order the H bus will arrive!
- Because we only covered char at this point in the semester all busses routes’ names are 1 character in length. We cannot have B-He or REXB routes.
See Rutgers bus routes below, where some are fictitious.
- A Route
- B Route
- C Route
- E Route
- F Route
- H Route
- L Route
- R Route
- X Route
Write the program BusStop.java that reads n char arguments from the command line.
- The first n-1 characters refer to the order in which the buses will arrive at the bus stop.
- The last char refers to the bus Serena is waiting for.
- The program displays the order in which the bus Serena is waiting for will arrive. If the bus Serena is waiting for does not stop at the bus stop then the program displays -1.
- To read the first character from the command line use:
- char c = args[0].charAt(0);
- To read the nth character from the command line use:
- char c = args[n-1].charAt(0);
- You will have to read an undetermined number of characters, so, you will need a loop to read all characters from the command line.
- Hint: Read the first n-1 values and store them into a char 1D array. Then, read the last char and search for that value in the array.
Example 1:
- java BusStop C L H H
- 3
The first bus to arrive is C, followed by L, then H. The program displays 3 because H is the third bus to arrive.
Example 2:
- java BusStop B R X B
- 1
The first bus to arrive is B, followed by R, then X. The program displays 1 because B is the first bus to arrive.
- Example 3:
- java BusStop B C E A B H L
- -1
The L bus does not stop at the bus stop, the program displays -1.
- Assume: all the inputs are char values.
- Problem by Manya Sood
- Staircase Builder (25 points). Let’s build a staircase with the help of a 2-dimensional (2D) array.
- Write a program, StaircaseBuilder.java, that reads 2 (two) integer arguments from the command line.
- the first argument refers to the dimension of a 2D array of characters, let us call this number d.
- the second argument refers to the total number of bricks to build a staircase.
- The program prints out a character 2D array of size d x d. Each cell of the character array contains either an X or an empty space.
- ‘X’ indicates a brick placement
- ‘ ‘ (one empty space) indicates that a brick was not placed in the cell not placed.
- The program also prints a statement indicating the number of bricks (an integer value) remaining after building the staircase.
- Note 1: Make sure bricks are placed from the bottom up. It is important to remember that the staircase must maintain an ascending order, where each step up, consists of one less brick placed than the previous step.
- Note 2: Make sure that “Bricks remaining: ” is written correctly. Computers are not smart and will mark it as wrong, therefore, penalizing you.
- Note 3: all array cells must have 1 character space ‘ ‘ except for the ‘X’ cells.
- Note 4: Make sure to use a char[][] array.
- Examples:
java StaircaseBuilder 3 6
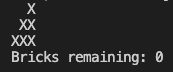
java StaircaseBuilder 12 98
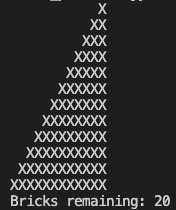
java StaircaseBuilder 5 10
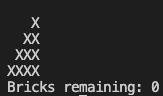
java StaircaseBuilder 5 7
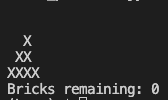
Hints: Place bricks one column at a time, starting at column zero.
- Start at column 0 (zero), place 1 brick.
- Then place an additional brick on every consecutive column.
- So it would 1 brick in the left most column, 2 in the next, 3 in the next, and 4 in the next, and so on.
- If the array is of size 0, no bricks can be placed.
Bellow are 2D arrays, from the above examples, that show the order in which bricks have been placed.
- the cells with numbers contain the ‘X’ character
- the cells that are empty contain the ‘ ‘ character
java StaircaseBuilder 3 6
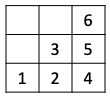
java StaircaseBuilder 12 98
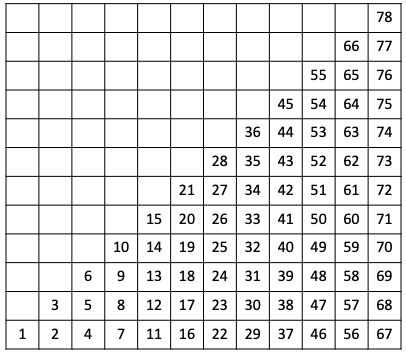
java StaircaseBuilder 5 10
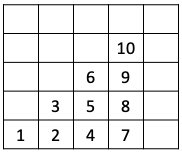
java StaircaseBuilder 5 7
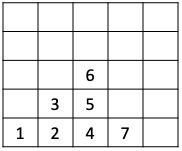
Every cell without a ‘X’ has a space.
In the example below the dots (‘.’) represent where the spaces are expected to be for java StaircaseBuilder 5 7
java StaircaseBuilder 5 7

Problem by Vidushi Jindal, Tanvi Yamarthy, and Maksims Kurjanovics Kravcenko
Before submission
Collaboration policy. Read our collaboration policy here.
Submitting the assignment. Submit BusStop.java and StaircaseBuilder.java separately via the web submission system called Autolab. To do this, click the Assignments link from the course website; click the Submit link for that assignment.
Getting help
If anything is unclear, don’t hesitate to drop by office hours or post a question on Piazza.
- Find instructors office hours here
- Find tutors office hours in Canvas -> Tutoring, RU CATS
- Find head TAs office hours here
- POST on Piazza in Canvas -> Piazza
- In addition to office hours we have the CAVE (Collaborative Academic Versatile Environment), a community space staffed with lab assistants which are undergraduate students further along the CS major to answer questions.