Conditionals and Loops – 40 course points
The purpose of this assignment is to practice conditionals and loops.
Refer to our Programming Assignments FAQ for instructions on how to install VScode, how to use the command line and how to submit your assignments.
Programming
Write 2 programs and submit on Autolab.
- WATCH this video: how to open the files on VSCode and how to submit into Autolab.
- We provide a zip file (find it under Buses on Autolab) containing Buses.java and DogWalk.java.
- For each problem UPDATE and SUBMIT the corresponding file.
Observe the following rules:
- DO NOT add any import statements
- DO NOT add the project statement
- DO NOT change the class name
- DO NOT change the headers of ANY of the given methods
- DO NOT add any new class fields
- DO NOT use System.exit()
- ONLY print the result as specified by each problem. Observe the examples’ output, display only what the problem is asking for.
- DO NOT print other messages, follow the examples for each problem.
- Buses (20 points). Write a program Buses.java that takes a 4-digit integer as a command-line argument and displays ERROR, LX, or H.
- You might have noticed that each Rutgers campus bus has its own four-digit number. Suppose that the sum:
- of an LX buses’ digits are always even.
- of an H buses’ digits are always odd.
- Write the program to read an integer input and display LX or H depending on which bus route it belongs to. The program displays ERROR if the input is negative and ends the program (does not ask for the input again).
- Note: You can use modulus to extract the last digit of a number.
- Assume: the input value used to test your program is a 4-digit integer.
java Buses 7302
LX
java Buses 6432
Hjava Buses -1432
ERROR
- Two-dimensional dog walk (20 points). Imagine that your dog’s collar has a tag that allows you to monitor how much exercise your dog is getting from their daily walk (safely with someone).
- This program will simulate the behavior of a dog walker, and your dog, moving randomly in the city.
- Imagine that the city is a two-dimensional grid of points.
- At each step, the walker randomly moves north, south, east, or west with probability equal to 1/4, independent of previous moves.
- Use Math.random() to generate a random number between 0.0 and 1.0
- Write a program DogWalker.java that takes an integer command-line argument n and simulates the motion of a walker randomly taking n steps.
- Print the location at each step (including the starting point), treating the starting point as the origin (0, 0).
- Also, print the square of the final squared Euclidean distance from the origin as double. The euclidean distance lets us you how far from the starting point (home) your dog is after n steps.
- Note 1: you do not need arrays for this problem, just keep track of the x and y coordinates during the random walk.
- Note 2: in the example below the walker takes 20 steps; there are 21 printed locations. The first location is the starting point (0,0).
java DogWalker 20
(0,0)
(0,1)
(-1,1)
(-1,2)
(0,2)
(1,2)
(1,3)
(0,3)
(-1,3)
(-2,3)
(-3,3)
(-3,2)
(-4,2)
(-4,1)
(-3,1)
(-3,0)
(-4,0)
(-4,-1)
(-3,-1)
(-3,-2)
(-3,-3)
Squared distance = 18.0
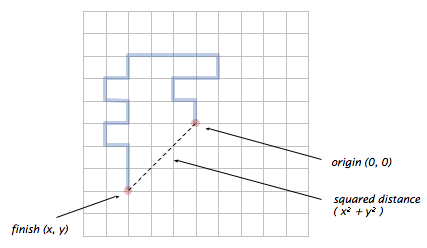
Before submission
Collaboration policy. Read our collaboration policy here.
Submitting the assignment. Submit Buses.java and DogWalker.java separately via the web submission system called Autolab. To do this, click the Assignments link from the course website; click the Submit link for that assignment.
Getting help
If anything is unclear, don’t hesitate to drop by office hours or post a question on Piazza.
- Find instructors office hours here
- Find tutors office hours in Canvas -> Tutoring, RU CATS
- Find head TAs office hours here
- POST on Piazza in Canvas -> Piazza
- In addition to office hours we have the CAVE (Collaborative Academic Versatile Environment), a community space staffed with lab assistants which are undergraduate students further along the CS major to answer questions.
Buses problem by Ayden Lyubimov